BitVMX off-chain communication system: Protocol Implementation and Practical Applications
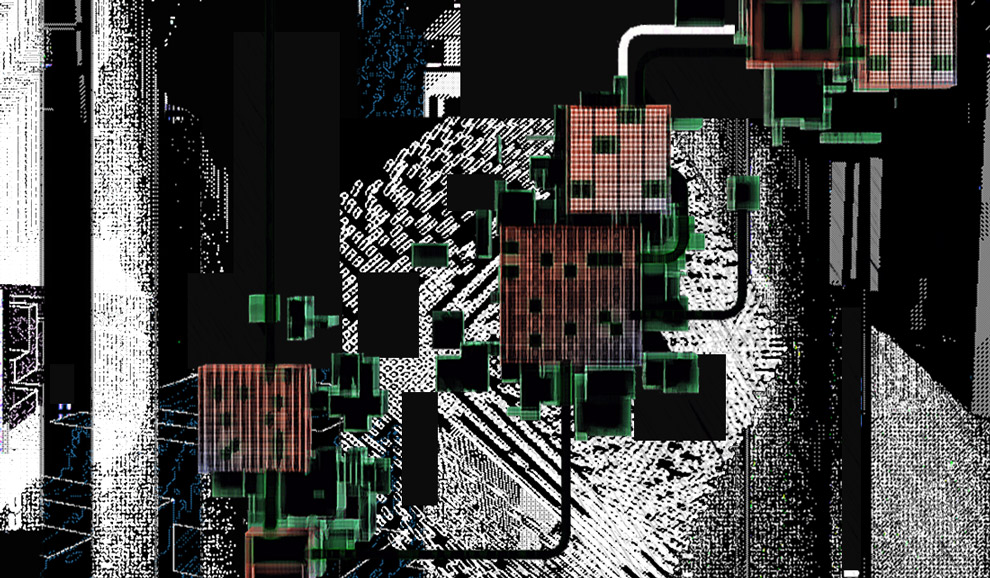
In the previous article, we introduced the BitVMX off-chain communication system, highlighting its decentralized P2P framework, the use of identity keys, and the Noise Protocol Framework for secure, encrypted connections. We also mentioned the system’s channel architecture, rate limiter, and other elements we will now explore in depth.
Channels
Now, we’ll take a closer look at the channel architecture within BitVMX. As explained previously, these channels handle both incoming and outgoing data, facilitating message exchanges between nodes while ensuring smooth interaction between the async and sync components of the system.
Within the system’s sync components, the receiver channel handles incoming messages and events from other nodes, while the sender channel is responsible for managing outgoing messages and directives.
The receiver channel can handle these types of data and events:
- Data: This handles messages received from other peers. Each message is delivered as a byte vector along with the peer ID of the sender. This ensures that the message origin is clearly identified.
- Status: This field is used to acknowledge receipt of messages from other peers. It contains a boolean value indicating whether the message was successfully received, along with the peer ID of the sender. This confirmation helps maintain message reliability.
- ConnectionLimit: This part of the channel receives notifications when a peer has reached its connection rate limit. It provides the peer ID or IP address of the affected peer, which is then automatically disconnected. Details about connection limits and rate-limiting mechanisms will be covered later.
- Error: If any error occurs during the P2P communication process, this field captures the error message, allowing for proper handling and error recovery.
On the other hand, the sender channel is responsible for directing outgoing messages and actions:
- Dial: This is used to initiate a connection with a new peer. It takes the IP address and peer ID of the target node, allowing for a direct dial to establish communication.
- SendMsg: This enables the sending of messages to an already connected peer. The message is sent as a byte vector, along with the peer ID of the recipient.
- DisconnectFrom: This function allows for disconnection from a specific peer. If a peer ID is provided, the system disconnects from that particular peer. If no peer ID is given, the system will disconnect from all peers, effectively shutting down the P2P async thread.
There is also a priority channel that monitors any dropped messages if the receiver buffer becomes full, whether due to temporary processing delays or an unusual influx of messages. By tracking dropped messages, the system can identify potential issues with processing delays or security threats, allowing for a timely response to prevent communication loss or resource exhaustion.
It is strongly recommended that the sync part of the system periodically check these channels for new messages or events. This helps ensure that no important data is left unattended for extended periods.
Rate limiter
As previously mentioned, the rate limiter is an essential functionality designed to protect nodes from certain types of repeated attacks or resource exhaustion attempts by other nodes. While external DoS (Denial of Service) attacks are typically mitigated at the network level through firewalls and other security measures, the rate limiter is particularly valuable for handling situations involving legitimate nodes that may exhibit malicious behavior.
The rate limiter operates by limiting the frequency with which specific actions can be performed by any given node, ensuring that no single peer can overwhelm another node's resources. It works by assigning a certain number of tokens to each node, with a predefined refill rate that gradually replenishes the token pool over time.
Every node, identified by its peer ID or IP address, has an associated token count. Whenever an action occurs that may indicate malicious behavior, a token is consumed. If a node runs out of tokens, it is automatically disconnected to prevent potential resource exhaustion attacks.
Here are some scenarios where tokens are spent:
- Failed Handshake Attempts: If a node tries to establish a connection but fails to complete the handshake, a token is consumed. This is a typical sign of either a misconfigured node or a potential attack attempt, where a malicious node could be repeatedly initiating connection requests without following through.
- IP and Peer ID Mismatch: When a node's peer ID doesn’t match the corresponding IP address during a connection attempt a token is spent. This mismatch could indicate an attempt at identity spoofing, where a malicious node tries to disguise itself as another node.
- Incorrect Message Format: If a node sends a message that does not adhere to the expected format, this is considered suspicious behavior. The message may be either intentionally malformed or corrupted. Each instance of receiving such a message results in the consumption of a token.
- Unanswered Acknowledgment Messages: Acknowledgment messages play a key role in confirming that communication between nodes is functioning correctly. If a node fails to respond to an acknowledgment request, this is considered problematic, and a token is spent. This helps protect against nodes that are improperly handling communication flows or intentionally ignoring critical requests.
- Others: Any activity that appears to aim at disrupting the smooth and direct flow of communication, or draining another node’s resources is flagged.
When a node exhausts all of its tokens, it is immediately disconnected to protect the other node from any potential attacks. This ensures that the malicious or faulty node can no longer attempt to drain resources or compromise the network's stability. However, legitimate nodes are unlikely to run out of tokens under normal circumstances, thanks to the rate limiter’s refill mechanism. Over time, tokens are replenished at a configurable rate, allowing normal, well-behaved nodes to continue operating smoothly even if they occasionally trigger the rate limiter.
The rate limiter’s parameters, such as the maximum number of tokens and the refill rate, are fully customizable during the configuration of the P2P module. By adjusting these settings, the system can adapt to different network environments and traffic patterns, offering both protection and performance optimization.
Usage
The general usage of the library is designed to be straightforward, allowing users to leverage the full functionality of the P2P protocol without needing to dive into async code. This makes it both user-friendly and flexible, as the library manages much of the complexity behind the scenes.
To initialize the channels and begin the P2P protocol, the user should simply call:

In this setup, P2p is the main object responsible for managing the P2P protocol. Its structure includes the sender channel, which is used to send messages or commands to the async part of the protocol; the receiver channel, which receives messages or status updates from the async part; a priority channel for handling critical information; and the runtime that supports the ongoing async processes within the P2P protocol. This single call initializes all channels and activates the async process, ensuring everything is ready to go.
Here, addr is the address to bind the node to, and privk is the private key for secure communication.
Communicating between the sync part of the application and the async P2P protocol is equally straightforward. To send data, you simply make use of the sender channel:

This sends a message or command to the async part of the protocol, where it will be processed. The data can be any byte array or specific instruction meant for P2P communication. Similarly, to receive messages or status updates from the protocol, you can use the receiver channel as follows:

The design ensures that both sending and receiving messages are handled efficiently without forcing the user to manage the underlying async code directly. Whether you’re maintaining connections, troubleshooting errors, or simply exchanging data with other peers, the library simplifies these processes, making P2P communication accessible and easy to implement
Future Work
One of the core goals for BitVMX is to be a highly flexible and adaptable framework, capable of supporting various communication models in different scenarios. While the current implementation focuses on secure and controlled P2P direct connections, future enhancements aim to address use cases where the network may need to accommodate a larger and more dynamic set of unknown nodes.
In such cases, a discovery protocol becomes essential, and this is where Distributed Hash Tables (DHT) come into play. A DHT is a decentralized network structure that plays a crucial role in distributed systems by eliminating single points of failure. It facilitates the distributed storage and retrieval of key-value pairs. In this network, each peer stores a portion of the DHT, which collectively forms a complete system for data lookup and routing.
When a new node wants to join a DHT network, it typically starts by connecting to one or more known bootstrap nodes. These bootstrap nodes serve as initial contact points but are not inherently superior to other nodes in the network. Once a node joins the DHT, it operates identically to all other nodes, with no special privileges or hierarchical status.
The strength of a DHT lies in its ability to manage large, dynamic networks efficiently, where nodes can join or leave without impacting the system’s overall stability. This characteristic makes it particularly suited for decentralized applications involving many participants.
However, there are challenges and potential vulnerabilities associated with DHT networks, particularly when it comes to bootstrap nodes. If all bootstrap nodes are compromised or malicious, the integrity of the network can be put at risk, as these nodes may mislead new participants or disrupt communication. To address this, future work will need to explore various strategies to mitigate this risk. Possible approaches could include dynamically managing the bootstrap nodes, improving detection mechanisms for compromised nodes, or introducing alternative methods to decentralize the discovery process, all aimed at minimizing the potential impact on network security.
There can also be issues after a node successfully integrates into the DHT. As part of future work, developing protocols to identify and isolate nodes that behave maliciously after gaining access to the network will be crucial. Additionally, mechanisms will need to be explored for allowing individual nodes to reject incoming connections from other peers that exhibit suspicious behavior, even if those peers are already part of the DHT.
While these challenges must be addressed, the benefits of implementing a DHT within BitVMX would be significant. It would greatly enhance the system’s capacity to handle large-scale P2P networks, enabling the platform to scale efficiently while maintaining its decentralized nature.
Summary
BitVMX is designed to create a flexible framework for blockchain bridges, oracles, and proof verifiers. A key component of BitVMX is its async P2P communication system, which uses unique identity keys to ensure secure interactions between nodes. The system prevents single points of failure by distributing responsibilities across a network of nodes, instead of relying on a centralized server.
To secure communication, multiple keys are employed: identity keys for unique identification, ephemeral keys for secure session establishment via Diffie-Hellman key exchanges, and static keys for authentication. The initial handshake protocol uses the Noise Protocol, allowing nodes to authenticate and establish encrypted communication channels to protect against attacks like MitM.
Rate-limiting is incorporated to guard against malicious nodes, ensuring that bad actors can't overwhelm the system. Channels are used to manage both external communication between nodes and internal communication within the system's async and sync components.
A pre-established allow list permits trusted nodes onto the network, with mechanisms for updates and error reporting. The message exchange process between nodes is well-defined and secure, with limitations on message size and response time.
Future enhancements aim to integrate Distributed Hash Tables (DHT) to accommodate larger, dynamic networks. DHT will improve the system's scalability but introduces new challenges, such as preventing malicious bootstrap nodes from compromising the network.